That's where Eclipse kicks in. With the CDT plug-in you can edit, compile and debug your C++ programs.
These are the required downloads (compiler, debugger and editor):
- MinGW and GDB installers from here (I've downloaded MinGW-5.1.3.exe from MinGW->Current section) and from here for the latter (I've downloaded gdb-6.3-2.exe from Snapshot section)
- EasyEclipse for C and C++ from here (I've downloaded release 1.2.1.1).
After creating a C++ project in Eclipse you will notice that there is warning complaining that cygpath is not found, it's a benign warning since we're not using Cygwin to build programs.
Eclipse expects that g++, make and gdb executables to be in PATH, since I don't like to pollute the global PATH environment variable with MinGW executables I've created a "launcher" for Eclipse that adds MinGW to PATH before running Eclipse.
Here is the code (eclipse.js):
var program = "eclipse.exe";
// Add the arguments
for (var i = 0; i < WScript.Arguments.length; ++i)
{
program += " " + WScript.Arguments.Item(i);
}
var shell = new ActiveXObject("WScript.Shell");
// Add mingw to path
var env = shell.Environment("PROCESS");
var path = "c:\\mingw\\bin;";
path += env("PATH");
env("PATH") = path;
// Execute the program and don't wait for completion
shell.Exec(program);
Copy the "launcher" to Eclipse directory (c:\Program Files\EasyEclipse for C and C++ 1.2.1.1) and use it from now on to start Eclipse.
We need one more step to get everything to work, change the C/C++ build command from make to mingw32-make (or rename mingw32-make it to make in c:\mingw\bin, if you want the eclipse project to be crossplatform) like in the picture bellow:
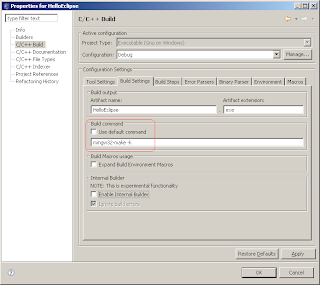
That was all. Now we have a full C++ IDE. Now we can develop console applications or Win32 C GUI applications. For C++ GUI applications we can use the free (GPL) Trolltech Qt library.